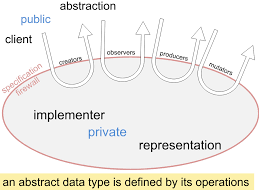
Data Structures and Algorithms (DSA) form the backbone of computer science. They provide the essential techniques for organizing and manipulating data efficiently, which is critical for building effective software. One of the fundamental concepts in DSA is the Abstract Data Type (ADT). This guide aims to introduce beginners to ADTs, their importance, and how they fit into the broader landscape of data structures and algorithms.
What is an Abstract Data Type?
An Abstract Data Type (ADT) is a model for a certain kind of data structure that provides a specific set of operations. It defines what operations can be performed on the data and what kind of data can be stored but does not specify how these operations are implemented. This abstraction allows programmers to focus on the high-level functionality without worrying about the underlying implementation details.
For example, consider a list. The ADT for a list might specify operations like adding an item, removing an item, and accessing an item by index. It does not concern itself with how these operations are carried out. The implementation details, such as whether the list is implemented as an array or a linked list, are left to the specific data structure.
Importance of Abstract Data Types
The concept of Abstract Data Types is crucial for several reasons:
-
Modularity: By separating the interface from the implementation, ADTs allow developers to change the implementation without affecting the rest of the program. This modularity leads to more maintainable and flexible code.
-
Reusability: ADTs provide a clear specification of the operations available on a data structure. This makes it easier to reuse code across different projects. Once an ADT is defined, its implementation can be reused as long as it conforms to the specified operations.
-
Abstraction: ADTs allow programmers to think about data structures at a higher level of abstraction. This simplifies the design process and helps in reasoning about the correctness and efficiency of algorithms.
Common Abstract Data Types
In a typical DSA course, you will encounter several common Abstract Data Types. Here are a few:
-
Stack: A stack is an ADT that follows the Last In, First Out (LIFO) principle. The primary operations are push (to add an element) and pop (to remove the most recently added element).
-
Queue: A queue is an ADT that follows the First In, First Out (FIFO) principle. The primary operations are enqueue (to add an element) and dequeue (to remove the oldest element).
-
List: A list is a sequence of elements with operations to add, remove, and access elements. Lists can be implemented as arrays or linked lists.
-
Tree: A tree is a hierarchical data structure with a root element and child elements. Common tree operations include insertion, deletion, and traversal.
-
Graph: A graph is a set of nodes connected by edges. Graphs can be used to represent networks, such as social networks or communication networks. Operations on graphs include adding/removing nodes and edges, and searching for paths.
Implementing Abstract Data Types
Implementing ADTs involves choosing appropriate data structures and algorithms to provide the required operations efficiently. For instance, a stack can be implemented using an array or a linked list. The choice of implementation can affect the performance of the operations.
Example: Implementing a Stack
Let’s consider a simple implementation of a stack using an array:
python
Copy code
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
return None
def is_empty(self):
return len(self.items) == 0
def peek(self):
if not self.is_empty():
return self.items[-1]
return None
# Usage
stack = Stack()
stack.push(1)
stack.push(2)
print(stack.pop()) # Output: 2
print(stack.peek()) # Output: 1
Learning Abstract Data Types
When learning ADTs in a DSA course, it is essential to understand both their theoretical and practical aspects. You will typically study the following:
-
Definitions and Operations: Learn the formal definitions of ADTs and the operations they support.
-
Implementation Techniques: Understand how to implement ADTs using various data structures.
-
Complexity Analysis: Analyze the time and space complexity of the operations to evaluate their efficiency.
-
Use Cases: Explore different scenarios where each ADT is applicable and beneficial.
Conclusion
Abstract Data Types are a foundational concept in Data Structures and Algorithms. They provide a way to define and work with data at a high level of abstraction, enabling more modular, reusable, and maintainable code. By mastering ADTs, you will be better equipped to tackle complex programming challenges and develop efficient software solutions.
Whether you are taking a DSA course or learning on your own, a solid understanding of Abstract Data Types will significantly enhance your programming skills. So dive in, explore the various ADTs, and practice implementing them to build a robust foundation in data structures and algorithms.