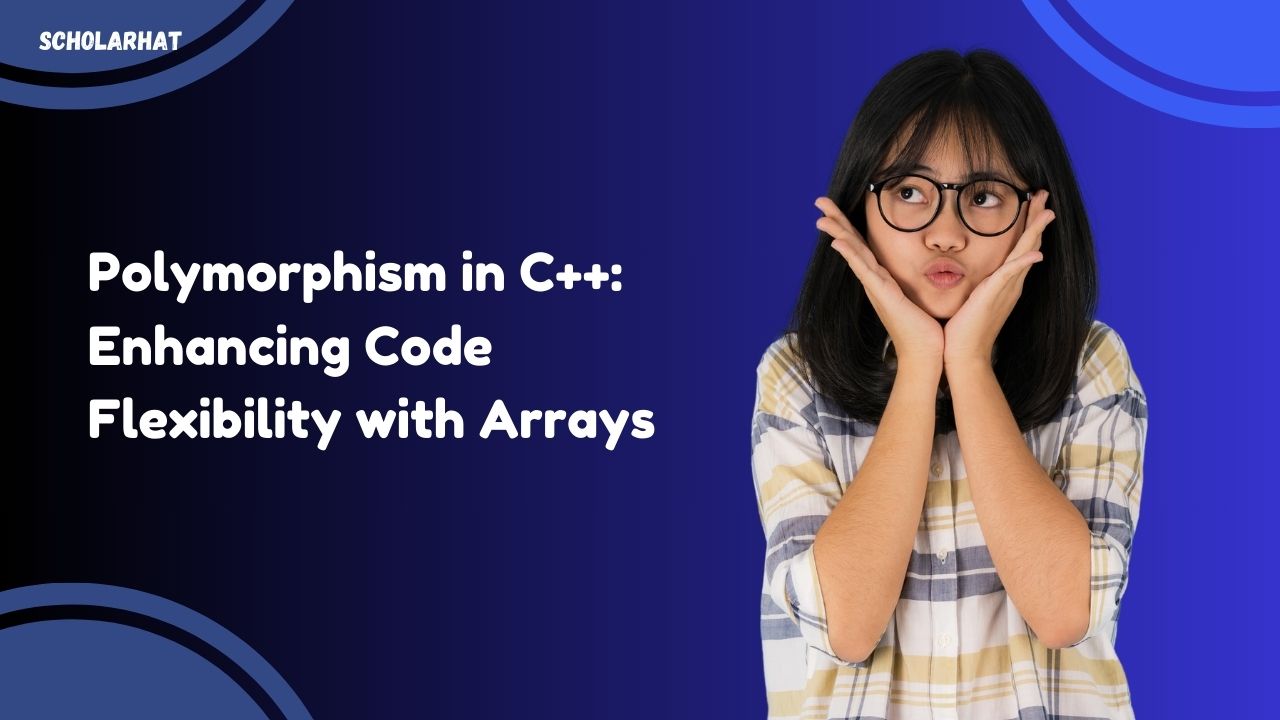
Polymorphism is a cornerstone of object-oriented programming in C++, enabling programmers to call the same function name but execute different functionalities. This powerful feature enhances code flexibility and readability, making it easier to manage and scale complex systems. In this article, we explore how polymorphism can be effectively used with arrays in C++, offering a deeper understanding of its applications and benefits.
Understanding Polymorphism in C++
Polymorphism in C++ can be achieved mainly in two ways: through compile-time polymorphism (also known as static polymorphism) using function overloading and templates, and runtime polymorphism (dynamic polymorphism) using virtual functions. Each method serves different purposes and provides various benefits, but the focus here will be on runtime polymorphism, which is particularly powerful when used with arrays of objects or pointers.
Runtime Polymorphism and Virtual Functions
Runtime polymorphism is implemented using virtual functions and inheritance. A virtual function is declared within a base class and overridden in a derived class. The decision to call the function defined in the base class or overridden in the derived class is made at runtime, hence the term “runtime polymorphism.”
Example of Runtime Polymorphism:
#include <iostream>
class Base {
public:
virtual void show() { // Virtual function
std::cout << “Base class show function called.” << std::endl;
}
};
class Derived : public Base {
public:
void show() override { // Override the base class function
std::cout << “Derived class show function called.” << std::endl;
}
};
int main() {
Base* b; // Base class pointer
Derived d; // Derived class object
b = &d;
b->show(); // Calls Derived’s show()
return 0;
}
This example demonstrates how a base class pointer can be used to call a derived class method, illustrating runtime polymorphism.
Using Polymorphism with Arrays
When dealing with arrays in C++, polymorphism can be utilized to handle a collection of objects from different but related classes uniformly. You can create an array of pointers to the base class that can hold addresses of objects of the derived classes.
Polymorphism with Array of Pointers
#include <iostream>
class Base {
public:
virtual void print() {
std::cout << “Base function” << std::endl;
}
virtual ~Base() {} // Virtual destructor for safe polymorphic use
};
class Derived1 : public Base {
public:
void print() override {
std::cout << “Derived1 function” << std::endl;
}
};
class Derived2 : public Base {
public:
void print() override {
std::cout << “Derived2 function” << std::endl;
}
};
int main() {
Base* array[2]; // Array of base class pointers
Derived1 d1;
Derived2 d2;
array[0] = &d1;
array[1] = &d2;
for(int i = 0; i < 2; ++i) {
array[i]->print(); // Calls print() according to the actual object type
}
return 0;
}
This setup demonstrates how an array of base class pointers can be used to invoke the appropriate print() function of each derived class without the need to know the type of object beforehand. For more on manipulating such structures, consider exploring C++ array techniques.
Benefits of Using Polymorphism with Arrays in C++
-
Flexibility: Allows the same array to store objects of different classes.
-
Scalability: Makes it easy to introduce new classes without altering the functionality of the existing classes or array handling code.
-
Maintainability: Enhances the manageability of the code, as modifications in the base class are automatically propagated to derived classes.
-
Code Reusability: Encourages more generic and reusable code elements, minimizing code duplication.
Conclusion
Polymorphism, particularly when combined with arrays in C++, offers a robust solution for designing flexible and scalable software systems. By understanding and implementing these concepts, developers can significantly improve the efficiency of their C++ applications. For a deeper dive into these topics, including sample code and best practices, exploring detailed discussions on polymorphism in C++ can provide valuable insights and advanced techniques.