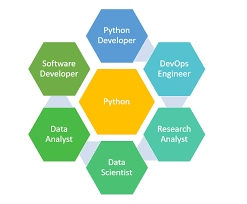
Python is a powerful and versatile programming language that is widely used in various fields, from web development to data science. For beginners, understanding how to implement basic mathematical concepts in Python can be an excellent way to grasp fundamental programming skills. In this article, we will delve into two essential programs: checking Armstrong numbers and prime numbers in Python.
Armstrong Number in Python
An Armstrong number, also known as a narcissistic number, is a number that is equal to the sum of its own digits each raised to the power of the number of digits. For example, 153 is an Armstrong number because 13+53+33=1531^3 + 5^3 + 3^3 = 15313+53+33=153. Let’s break down how to check if a number is an Armstrong number in Python.
Armstrong Number Program
Here is a simple Python program to check if a given number is an Armstrong number:
python
Copy code
# Function to check if a number is an Armstrong number
def is_armstrong_number(num):
# Convert the number to a string to get the number of digits
num_str = str(num)
num_digits = len(num_str)
# Calculate the sum of each digit raised to the power of num_digits
sum_of_powers = sum(int(digit) ** num_digits for digit in num_str)
# Check if the sum of powers is equal to the original number
return sum_of_powers == num
# Input number
number = int(input(“Enter a number: “))
# Check and print result
if is_armstrong_number(number):
print(f”{number} is an Armstrong number.”)
else:
print(f”{number} is not an Armstrong number.”)
In this program:
-
We define a function is_armstrong_number that takes a number as input.
-
The number is converted to a string to easily count the digits.
-
We calculate the sum of each digit raised to the power of the total number of digits.
-
The function returns True if this sum equals the original number, indicating it is an Armstrong number.
Prime Number in Python
A prime number is a natural number greater than 1 that has no positive divisors other than 1 and itself. For instance, 5 is a prime number because its only divisors are 1 and 5. Checking for prime numbers is a common programming exercise that helps beginners understand loops and conditionals.
Prime Number Program
Here is a straightforward prime number Python program to check if a given number is a prime number:
python
Copy code
# Function to check if a number is a prime number
def is_prime_number(num):
# Prime numbers are greater than 1
if num <= 1:
return False
# Check for factors from 2 to the square root of num
for i in range(2, int(num**0.5) + 1):
if num % i == 0:
return False
return True
# Input number
number = int(input(“Enter a number: “))
# Check and print result
if is_prime_number(number):
print(f”{number} is a prime number.”)
else:
print(f”{number} is not a prime number.”)
In this program:
-
We define a function is_prime_number that takes a number as input.
-
We immediately return False if the number is less than or equal to 1, as prime numbers are greater than 1.
-
We check for divisors from 2 up to the square root of the number. If any divisor is found, the number is not prime.
-
The function returns True if no divisors are found, indicating the number is prime.
Conclusion
Understanding how to implement basic mathematical checks in Python, such as determining Armstrong numbers and prime numbers, is an excellent way to build foundational programming skills. The concept of an Armstrong number in Python showcases the use of loops and mathematical operations, while checking for prime numbers in Python introduces beginners to conditional statements and efficient algorithms. With these simple yet powerful examples, beginners can start to appreciate the elegance and power of Python programming. Happy coding!