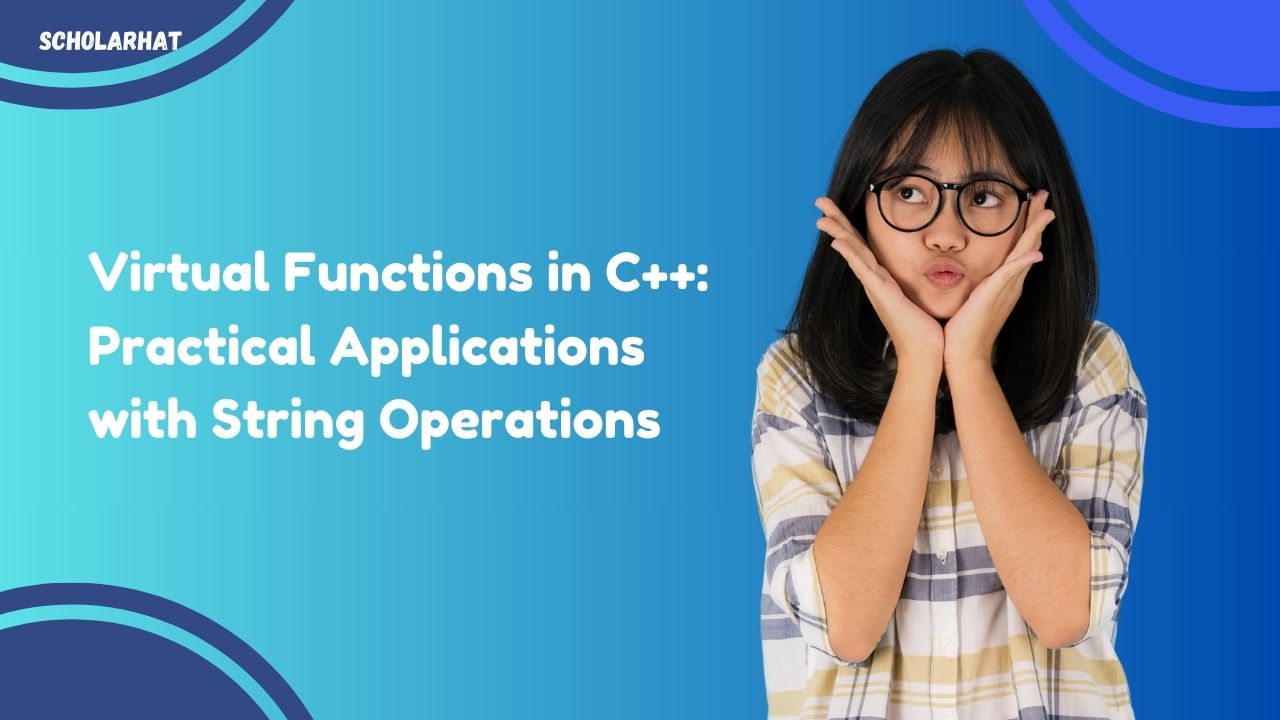
Virtual functions are a core concept in C++ object-oriented programming, providing a mechanism for runtime polymorphism. This allows for more flexible and manageable code, especially when dealing with a hierarchy of classes. In this article, we explore practical applications of virtual functions in C++, specifically how they can be leveraged in conjunction with string operations, an essential aspect of almost any C++ program.
Understanding Virtual Functions
A virtual function in a base class is declared with the virtual keyword and is intended to be overridden in any derived class. The purpose of virtual functions is to ensure that the correct function is called for an object, regardless of the type of reference (or pointer) used to call the function. This is crucial for operations where behavior might differ significantly between base and derived classes.
Syntax and Basic Concept
Here is a basic example of how virtual functions are declared and used in C++:
#include <iostream>
using namespace std;
class Base {
public:
virtual void display() { // Virtual function
cout << “Display base class” << endl;
}
virtual ~Base() {} // Virtual destructor to ensure proper cleanup
};
class Derived : public Base {
public:
void display() override { // Override the base class function
cout << “Display derived class” << endl;
}
};
void printInfo(Base& obj) {
obj.display(); // Polymorphic call
}
In this example, display() is a virtual function. This setup ensures that the correct display() method is called, depending on the actual object type, not the type of reference.
Virtual Functions and String Operations
Consider a scenario where different classes deal with different types of string manipulations. Using virtual functions can simplify managing these operations across various object types. Below is a practical example using string operations:
#include <iostream>
#include <string>
using namespace std;
class StringManipulator {
public:
virtual string transform(string input) = 0; // Pure virtual function
virtual ~StringManipulator() {}
};
class Reverser : public StringManipulator {
public:
string transform(string input) override {
reverse(input.begin(), input.end());
return input;
}
};
class Capitalizer : public StringManipulator {
public:
string transform(string input) override {
for (char& c : input) {
c = toupper(c);
}
return input;
}
};
void displayTransformation(StringManipulator* obj, const string& data) {
cout << “Transformed string: ” << obj->transform(data) << endl;
}
In this example:
-
StringManipulator is an abstract class with a pure virtual function transform().
-
Reverser and Capitalizer are derived classes that implement the transform() function differently: one reverses a string, while the other capitalizes it.
-
The displayTransformation() function demonstrates polymorphic behavior, calling the correct transform() method depending on the object passed.
Benefits of Using Virtual Functions with String Operations
-
Flexibility: Easily introduce new string manipulation strategies by simply adding new classes.
-
Maintainability: Central changes in the base class or function interface do not require changes in client code.
-
Code Reusability: Share and reuse code, such as the displayTransformation() function, across different string manipulation strategies.
Practical Use Cases
-
Text Editors: Handling different formatting or parsing strategies for text.
-
Communication Systems: Encoding and decoding messages differently depending on the protocol or user settings.
-
Data Processing Applications: Applying different data cleanup rules based on the data source or type.
Conclusion
Virtual functions are a powerful feature in C++ that allow developers to design and implement software that is both flexible and easy to manage. When combined with string operations, virtual functions enable the construction of systems that can easily adapt to new requirements with minimal changes to existing code.
For developers working with C++, understanding and applying virtual functions can lead to cleaner, more efficient, and scalable code solutions. For further exploration of string handling and virtual functions in C++, reviewing detailed resources on virtual function in C++ and C++ string operations is highly recommended.